- Published on
Spring Boot Job Interview Questions and Answers
- Authors
- Name
- Luis Carbonel
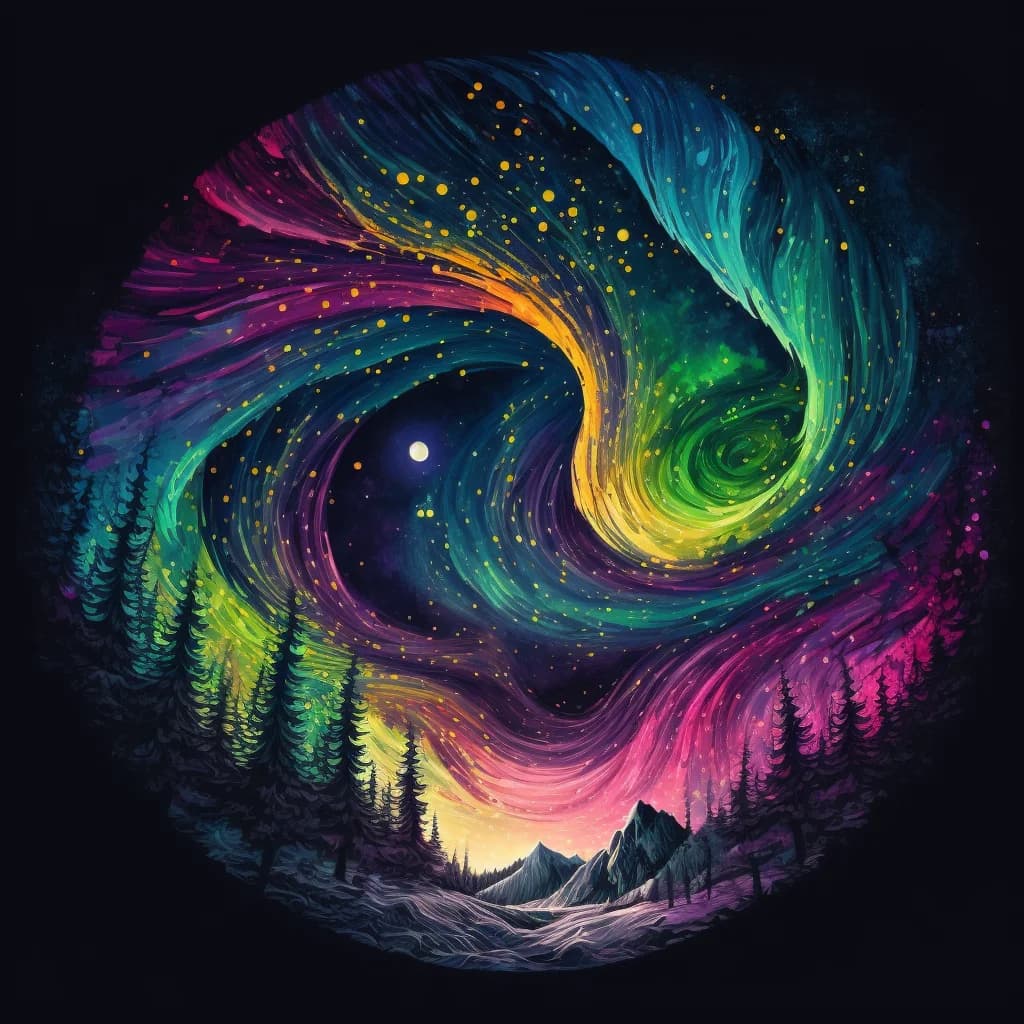
Spring Boot is a popular framework that simplifies the development of web applications by providing out-of-the-box features and functionalities. Many companies use Spring Boot as their primary development framework, and as a result, job interviews for Spring Boot developers are common. Also, many times Spring Boot questions are usually linked to Java job interview questions. In this technical post, we will discuss some of the frequently asked Spring Boot job interview questions, along with their answers
What is Spring Boot, and How Does it Differ from Spring Framework?
Spring Boot is a framework that builds upon the Spring Framework, streamlining the development of Spring-based applications. It reduces the need for boilerplate code and simplifies application configuration by offering pre-configured features and functionality. Spring Boot provides numerous out-of-the-box features, such as embedded servers, database connectivity, security, and more, enabling developers to create production-ready applications rapidly.
Understanding Dependency Injection in Spring Boot
Dependency injection is vital in Spring Boot, promoting the "inversion of control" principle. It allows objects to depend on others without knowing how they are created. Spring Boot implements this using:
Constructor Injection: Preferred for injecting dependencies via constructors, ensuring all dependencies are set during object creation.
Setter Injection: Useful for optional or numerous dependencies, injected via setter methods.
Field Injection: Dependencies are directly injected into fields. While concise, it may lead to less testable code and complexity.
Constructor injection is the recommended approach for dependency injection in Spring Boot, as it offers better immutability and testability.
Let's look at an example of constructor injection in Spring Boot:
@Service
public class MyService {
private final MyDependency myDependency;
public MyService(MyDependency myDependency) {
this.myDependency = myDependency;
}
//...
}
In this example, the MyService
class depends on MyDependency
, which is injected via the constructor. This approach ensures that MyService
always has a valid dependency, and it's easy to test.
To make this work, we need to tell Spring Boot how to create and inject the MyDependency object. We can do this by annotating the MyDependency class with @Component
or by defining a bean in our application context as shown in the following example:
@Configuration
public class AppConfig {
@Bean
public MyDependency myDependency() {
return new MyDependency();
}
}
In this example, we define a bean named myDependency
of type MyDependency
in the AppConfig
class. Whenever Spring Boot needs to create an instance of MyService, it will automatically inject the myDependency
bean into the constructor.
The Spring Bean Lifecycle
When working with Spring Boot, beans go through a well-defined lifecycle:
Bean Instantiation: On startup, Spring Boot reads configuration metadata and creates instances of defined beans. This process includes setting specified bean properties.
Dependency Injection: After instantiation, Spring Boot injects dependencies into the beans, satisfying any requirements they may have on other beans.
Aware Interfaces: Spring Boot checks if beans implement certain interfaces. If they do, relevant dependencies are injected, and callback methods are invoked. Examples include
ApplicationContextAware
,EnvironmentAware
, andResourceLoaderAware
.Bean Initialization: Once all dependencies are injected, Spring Boot calls methods annotated with
@PostConstruct
. These methods allow beans to perform initialization tasks.Bean Usage: Beans are now fully initialized and ready for use. Other components in the application can inject instances of these beans as needed.
Bean Destruction: When the application shuts down, Spring Boot calls methods annotated with
@PreDestroy
. These methods facilitate necessary cleanup tasks before the bean is removed from memory.
Principal Annotations in Spring Boot
Spring Boot uses various annotations to simplify configuration:
@SpringBootApplication
: Marks the main class of a Spring Boot application, enabling auto-configuration and component scanning.@Autowired
: Injects a bean into a Spring-managed component.@Component
: Identifies a class as a Spring-managed component, enabling auto-detection and wiring of Spring beans.@Configuration
: Marks a class as a configuration class, used to define beans and their dependencies.@EnableAutoConfiguration
: Enables Spring Boot’s autoconfiguration feature, which configures applications based on dependencies in the classpath.@RestController
: Flags a class as a RESTful controller, combining@Controller
and@ResponseBody
annotations.@GetMapping
,@PostMapping
,@PutMapping
,@DeleteMapping
: Map HTTP requests to methods in a controller.
@Component
, @Repository
, @Service
, and @Controller
Understanding the Differences: All four annotations can be used interchangeably, depending on the intended purpose and developer preference:
@Component: Marks a class as a Spring-managed component. Useful for generic utility functions.
@Repository: Identifies a class as providing a persistence layer service, often used for data access and persistence.
@Service: Flags a class as providing business logic services, typically high-level services for specific domains or user stories.
@Controller: Marks a class as a Spring-managed controller for handling web requests, handling HTTP requests and returning HTTP responses.
What is Actuator in Spring Boot?
Actuator is a Spring Boot subproject that offers production-ready features like health checks, metrics, and monitoring. It exposes endpoints via HTTP or JMX, providing insights into an application’s internal state, including health, metrics, environment, and beans.
To expose a basic health endpoint, you can configure it in your application.properties
file:
management.endpoints.web.exposure.include=health
This enables the health endpoint, which offers information about your application’s health. By default, it returns "UP" if the application is running smoothly, but you can customize the health check logic to include additional checks like database connectivity or external services.
Access the health endpoint with a GET request to http://localhost:8080/actuator/health
(assuming your app runs on port 8080). The response is a JSON object containing health status information.
Configuring Database Access in Spring Boot
In Spring Boot, configuring database access is straightforward:
Add the database driver and Spring Data JPA dependency to your project's build file (e.g.,
pom.xml
for Maven orbuild.gradle
for Gradle).Configure the database connection properties in
application.properties
orapplication.yml
. Example for MySQL:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=password
spring.jpa.hibernate.ddl-auto=update
Create an entity class representing a database table with appropriate annotations (e.g.,
@Entity
,@Id
,@GeneratedValue
,@Column
).Create a repository interface extending
JpaRepository
with CRUD and custom methods.Inject the repository into your service or controller using
@Autowired
and use its methods for database interaction.
Optimistic Locking in Spring Boot
Optimistic locking in Spring Boot prevents multiple users from concurrently updating the same data. It works by allowing multiple users to read data simultaneously. When a user attempts to update the data, Spring Boot checks whether another user has modified it since the last read. If so, the update is rejected, and the user must re-read and retry.
Optimistic locking is implemented using the @Version
annotation on an entity class, which increments a version field upon each update. When saving or updating the entity, Spring Boot checks if the versions match. If not, it indicates that another user updated the data, and the update is rejected.
Here's a simple example in Spring Boot:
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@Version
private Long version;
// getters and setters
}
@Service
public class EmployeeService {
@Autowired
private EmployeeRepository employeeRepository;
@Transactional
public Employee updateEmployee(Long id, String newName) {
Employee employee = employeeRepository.findById(id).orElseThrow(EntityNotFoundException::new);
employee.setName(newName);
return employeeRepository.save(employee);
}
}
@RestController
public class EmployeeController {
@Autowired
private EmployeeService employeeService;
@PutMapping("/employees/{id}")
public ResponseEntity<Employee> updateEmployee(@PathVariable Long id, @RequestBody String newName) {
try {
Employee updatedEmployee = employeeService.updateEmployee
(id, newName);
return ResponseEntity.ok(updatedEmployee);
} catch (OptimisticLockingFailureException e) {
return ResponseEntity.status(HttpStatus.CONFLICT).build();
}
}
}
In this example, @Version
is used for optimistic locking, and a conflict is handled if updates conflict.
Conclusion
In conclusion, Spring Boot is a powerful and versatile framework for building robust and scalable applications. In a job interview for a Spring Boot developer position, it’s essential to have a strong understanding of the framework’s concepts, features, and best practices.