- Published on
Most used Kotlin frameworks
- Authors
- Name
- Luis Carbonel
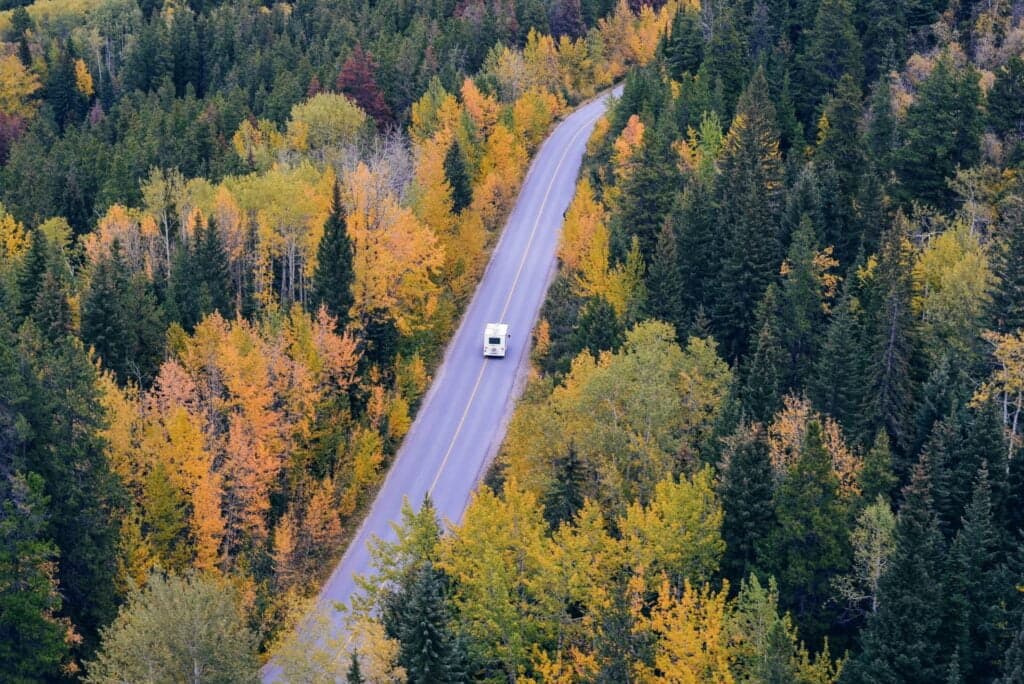
Kotlin has emerged as a popular programming language for developing backend applications due to its concise syntax, type safety, null safety, and interoperability with Java. Kotlin provides a range of frameworks that enable developers to build robust and scalable backend applications. In this post, we will discuss some of the most used Kotlin frameworks.
Spring Boot
Spring Boot is a popular Java-based framework for building enterprise applications, and it has strong support for Kotlin. Kotlin developers can leverage the Spring Boot framework to build robust, scalable, and modular backend applications. Spring Boot provides various features like dependency injection, auto-configuration, and support for various databases and messaging services. It is also known for its excellent community support, which ensures that developers can easily find answers to their queries.
Spring boot provides two ways to build a server with Kotlin, you can use Spring Web or if you want a reactive API you can use Spring WebFlux. Spring Web uses a traditional servlet-based programming model, it is not designed to handle large numbers of concurrent requests. Spring WebFlux, however, is specifically designed to handle high concurrency, making it a better choice for applications that need to handle large numbers of concurrent requests.
Here are two examples, one using Spring Web and the other using Spring WebFlux with Kotlin:
Spring Web
import org.springframework.web.bind.annotation.GetMapping
import org.springframework.web.bind.annotation.RestController
@RestController
class HelloController {
@GetMapping("/hello")
fun hello(): String {
return "Hello, World!"
}
}
In the above code, we are using Spring Web to create a simple RESTful API that responds with “Hello, World!” when accessed at the “/hello” path.
Spring WebFlux
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.flow
import org.springframework.web.bind.annotation.GetMapping
import org.springframework.web.bind.annotation.RestController
@RestController
class HelloController {
@GetMapping("/hello")
suspend fun hello(): Flow<String> = flow {
emit("Hello, World!")
}
}
In this example, we are using Spring WebFlux with coroutines to create a simple RESTful API that responds with “Hello, World!” when accessed at the “/hello” path. We are also using the Flow type to represent the asynchronous response. We can use coroutines with Spring WebFlux by returning a Flow from the endpoint function, which will automatically be converted to a reactive stream.
We can also make use of Spring Reactor, but I recommend using what we saw in the previous example using suspend and Flow.
import org.springframework.web.bind.annotation.GetMapping
import org.springframework.web.bind.annotation.RestController
import reactor.core.publisher.Mono
@RestController
class HelloController {
@GetMapping("/hello")
fun hello(): Mono<String> {
return Mono.just("Hello, World!")
}
}
Ktor
Ktor is a lightweight and flexible Kotlin-based framework for building asynchronous servers and clients. It is built on top of the Kotlin coroutines and offers features like routing, client and server-side HTTP APIs, authentication, and WebSocket support. Ktor is easy to use and configure, making it a popular choice for developing web applications, microservices, and RESTful APIs.
In the following example, we are using Ktor to create a simple RESTful API that responds with “Hello, World!”
import io.ktor.application.*
import io.ktor.response.*
import io.ktor.routing.*
import io.ktor.server.engine.*
import io.ktor.server.netty.*
fun main(args: Array<String>) {
embeddedServer(Netty, port = 8080) {
routing {
get("/") {
call.respondText("Hello, World!")
}
}
}.start(wait = true)
}
Vert.x
Vert.x is a lightweight and modular framework for building reactive applications. It is built on top of the Kotlin coroutines and offers features like routing, client and server-side HTTP APIs, authentication, and WebSocket support.
Vert.x is easy to use and configure and offers features like event-driven programming, non-blocking I/O, distributed event bus, and support for different messaging protocols like MQTT and AMQP. It is also modular, enabling developers to choose the components they need and build custom applications.
In the following example, we are using Vert.x to create a simple RESTful API that responds with “Hello, World!”
import io.vertx.core.Vertx
fun main() {
val vertx = Vertx.vertx()
vertx.createHttpServer().requestHandler { req ->
req.response().end("Hello, world!")
}.listen(8080)
}
In this example, we are using Vert.x to create an HTTP server that listens on port 8080 and responds with “Hello, world!” to any incoming request. We are using the Vertx class to create an instance of the Vert.x runtime, and the createHttpServer() method to create an HTTP server. The requestHandler() method is used to handle incoming requests, and the end() method is used to send a response. Finally, the listen() method is used to start the server and listen for incoming requests.
Micronaut
Micronaut is a modern, lightweight, and modular framework for building microservices and serverless applications. It is built on top of the Kotlin coroutines and offers features like dependency injection, auto-configuration, and support for various databases and messaging services. Micronaut is easy to use and configure, making it a popular choice for developing microservices and serverless applications.
In the following example, we are using Micronaut to create a simple RESTful API that responds with “Hello, World!”
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
@Controller("/hello")
class HelloController {
@Get("/")
fun hello(): String {
return "Hello, World!"
}
}
Koin
Koin is a lightweight and pragmatic dependency injection framework for Kotlin. It enables developers to manage the lifecycle of their components and provides a simple and elegant way to declare dependencies. Koin is easy to learn and use, making it a popular choice for building small to medium-sized backend applications.
import org.koin.core.context.startKoin
import org.koin.core.module.Module
import org.koin.dsl.module
import org.koin.java.KoinJavaComponent.inject
class MyService(val message: String)
val myModule: Module = module {
single { MyService("Hello, World!") }
}
fun main(args: Array<String>) {
val koin = startKoin { modules(myModule) }.koin
val myService: MyService by inject(MyService::class.java)
println(myService.message)
}
In the above code, we are using Koin to create a simple service that prints “Hello, World!” to the console when run. We need to start Koin with our application. Just call the startKoin() function in the application’s main entry point.
Frameworks links
Conclusion
In conclusion, Kotlin provides a range of backend frameworks that enable developers to build robust, scalable, and maintainable applications. Each of the frameworks discussed above has its strengths and weaknesses, and developers should choose the one that best fits their needs and requirements.
I hope you enjoyed this article, if you have any questions or comments, please leave them below.